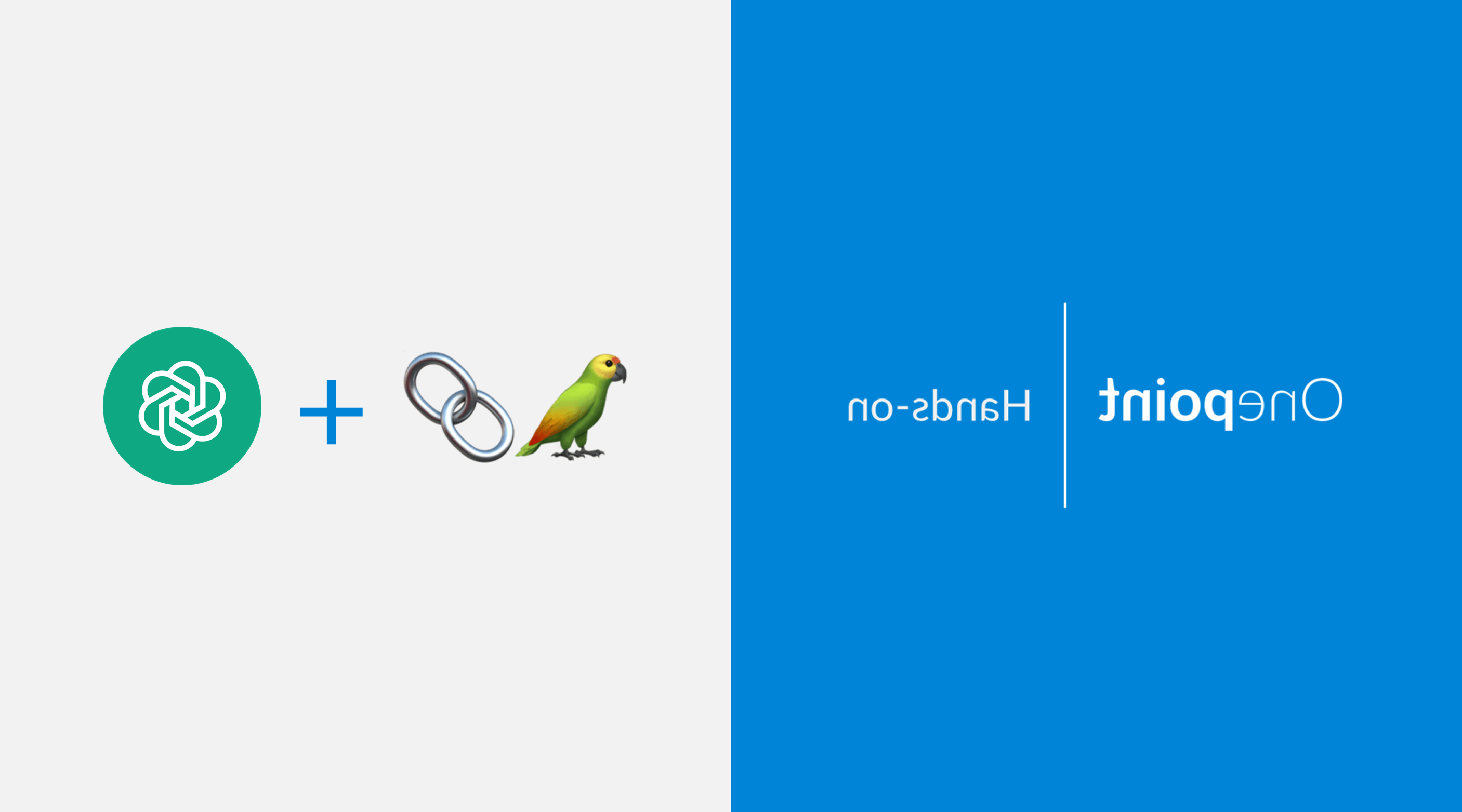
在这个故事中,我们将描述如何使用 LangChain (v. 0.0.190) with ChatGPT under the hood. 这个故事是一个 previous story on Medium 是建立在那个故事的思想基础上的.
LangChain has a set of foundational chains:
- LLM:一个带有提示模板的简单链,可以处理多个输入.
- RouterChain:使用大型语言模型(LLM)选择最合适的处理链的网关.
- Sequential:按顺序处理输入的一系列链. 这意味着链中第一个节点的输出, 成为第二个节点的输入和第二个节点的输出, 第三个输入,以此类推.
- Transformation:一种链,允许Python函数调用可定制的文本操作.
A Complex Workflow
在这个故事中,我们将使用所有基础链来创建以下简单的命令行应用程序工作流:
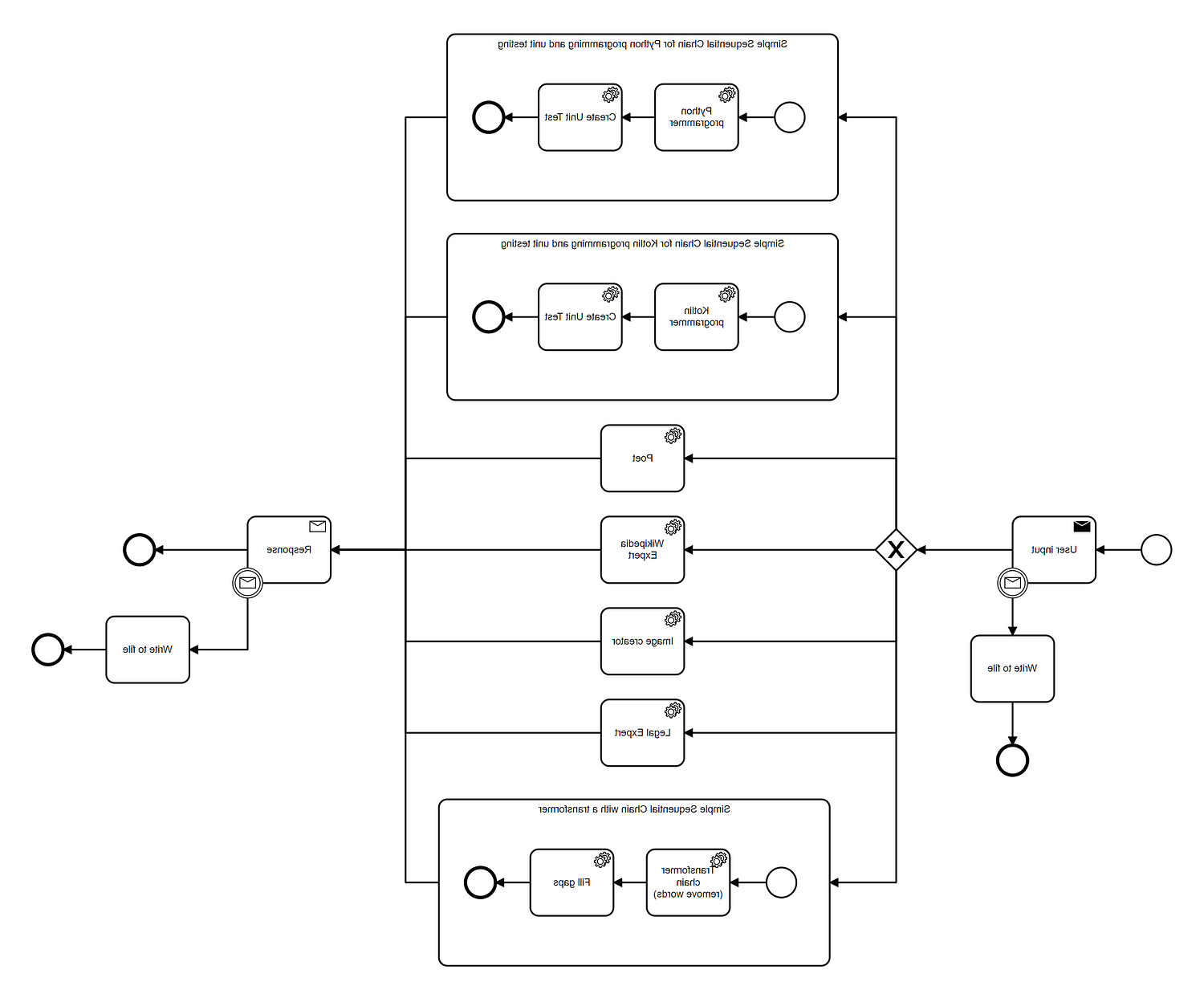
该流程执行以下步骤:
- Receive the user input
- 输入通过回调写入文件.
- 路由器从五个选项中选择最合适的链:
Python程序员(使用顺序链提供解决方案和单元测试)
Kotlin程序员(使用顺序链提供解决方案和单元测试)
- Poet(提供单个响应的简单LLMChain)
-维基百科专家(简单的LLMChain)
-图形美工(简单的LLMChain)
-英国、美国法律专家(simple LLMChain)
-词间隙填充器(包含转换输入并填充空白的顺序链) - 大语言模型(LLM)响应.
- 输出再次通过回调写入文件.
正如你所看到的,主路由器链会触发简单的LLMChain,但也会触发SimpleSequentialChain.
Flow Implementation
我们已经在这个Github存储库中发布了上述流程的Python实现:
GitHub - gilfernandes/complex_chain_playground:游乐场项目作为一个例子…
Playground项目作为一个复杂的LangChain工作流的例子- GitHub…
如果您想使用它,您可以克隆存储库,然后使用 Conda with Mamba.
下面是我们用来安装必要库的脚本:
Conda create——name langchain2 python=3.10
激活langchain2
Conda安装-c Conda -forge mamba
mamba install openai
mamba install langchain
Mamba安装prompt_toolkit
You will need a ChatGPT key 安装在您的环境中以使脚本工作.
在Linux中,您可以执行像这样的脚本来设置 ChatGPT key:
export OPENAI_API_KEY=
然后你可以激活Conda环境并运行脚本:
激活langchain2
python ./lang_chain_router_chain.py
Example Output
我们执行了带有一些问题的脚本,并捕获了一个 transcript in this file.
下面是一些我们用作输入的提示和相应的被触发的代理:
你能帮我把这篇课文中的单词填上吗? 强化学习(RL)是机器科学的一个领域
学习关注的是智能代理应该如何在环境中采取行动
为了最大化累积奖励的概念.
强化学习是三种基本的机器学习范式之一,
还有监督学习和非监督学习.
- word filler
删除每三个单词,然后填补空白
英国和美国法律体系的主要区别是什么
就遗产税而言?
- legal expert
产生一个解释与法律的比较
你能写一个返回日期列表的Python函数吗
between two dates?
- python programmer
生成代码,然后进行单元测试
你能写一个Python函数来实现Levenshtein距离吗
between two words?
- python programmer
生成代码,然后进行单元测试
你能写一个Kotlin函数转换两个日期在ISO格式
(like e.g. '2023-01-01')转换为LocalDate,然后计算天数
between both?
- kotlin programmer
生成代码,然后进行单元测试
你能写一首十大网博靠谱平台软件开发乐趣的诗吗
在英国的乡村?
- poet
generates a poem:
在代码的领域里,逻辑是存在的
在青翠的田野里,软件栖息.
在那里,字节和比特随着微风翩翩起舞,
在英国乡村,程序员可以轻松地工作.
你能用s型函数的输出生成一个图像吗
and its derivative?
- graphical artist
生成SVG图像(不是很准确)
你能给我解释一下量子计算中量子比特的概念吗?
- wikipedia expert
生成对主题的适当解释
Implementation Details
该项目包含一个主脚本,用于设置链并执行它们: complex_chain.py. 项目里还有其他文件,比如 FileCallbackHandler.py 这是一个用于将模型输入和输出写入HTML文件的回调处理程序的实现.
我们现在要关注的是 complex_chain.py.
complex_chain.py sets up the model first:
class Config():
model = 'gpt-3.5-turbo-0613'
llm = ChatOpenAI(model=model, temperature=0)
它声明了的一个特殊变体 langchain.chains.router.MultiPromptChain,因为我们不能把它们一起使用 langchain.chains.SimpleSequentialChain:
类MyMultiPromptChain (MultiRouteChain):
"""一个多路由链,它使用LLM路由链来选择提示."""
router_chain: RouterChain
用于决定目标链及其输入的链."""
destination_chains: Mapping[str, Union[LLMChain, SimpleSequentialChain]]
名称到候选链的映射,输入可以路由到候选链."""
default_chain: LLMChain
"""当路由器没有将输入映射到目的地时使用的默认链."""
@property
def output_keys(self) -> List[str]:
return ["text"]
然后生成所有链(包括默认链)并将它们添加到列表中:
def generate_destination_chains ():
"""
创建具有不同提示模板的LLM链列表.
请注意,有些链是顺序链,用来生成单元测试.
"""
prompt_factory = PromptFactory()
destination_chains = {}
对于prompt_factory中的p_info.prompt_infos:
name = p_info['name']
Prompt_template = p_info[' Prompt_template ']
chain = LLMChain(
llm=cfg.llm,
提示= PromptTemplate(模板= prompt_template input_variables =(“输入”)),
output_key='text',
回调函数= [file_ballback_handler]
)
如果名称不在prompt_factory中.programmer_test_dict.keys() and name != prompt_factory.word_filler_name:
Destination_chains [name] = chain
Elif name == prompt_factory.word_filler_name:
transform_chain = transform_chain
input_variables =(“输入”), output_variables =(“输入”), 变换= create_transform_func (3), 回调函数= [file_ballback_handler]
)
destination_chains[name] = SimpleSequentialChain()
chains=[transform_chain, chain], verbose=True, output_key='text', 回调函数= [file_ballback_handler]
)
else:
# Normal chain用于生成代码
生成单元测试的附加链
模板= prompt_factory.programmer_test_dict[名称]
prompt_template = PromptTemplate(input_variables =(“输入”), template=template)
test_chain = LLMChain(llm=cfg . cfg).Llm, prompt=prompt_template, output_key='text', 回调函数= [file_ballback_handler])
destination_chains[name] = SimpleSequentialChain()
chains=[chain, test_chain], verbose=True, output_key='text', 回调函数= [file_ballback_handler]
)
default_chain = ConversationChain(llm=cfg).llm, output_key="text")
return prompt_factory.prompt_info, destination_chains, default_chain
它建立了路由器链:
Def generate_router_chain(prompt_info, destination_chains, default_chain):
"""
根据提示信息生成路由器链.
:param prompt_info上面生成的提示信息.
:param destination_chains使用不同提示模板的LLM链
default_chain默认链
"""
目的地= [f"{p['name']}: {p['description']}" for p in prompt_info]
destinations_str = '\n'.join(destinations)
router_template = MULTI_PROMPT_ROUTER_TEMPLATE.格式(目的地= destinations_str)
router_prompt = PromptTemplate(
模板= router_template,
input_variables =(“输入”),
output_parser = RouterOutputParser ()
)
router_chain = LLMRouterChain.from_llm(cfg.llm, router_prompt)
multi_route_chain = MyMultiPromptChain(
router_chain = router_chain,
destination_chains = destination_chains,
default_chain = default_chain,
verbose=True,
回调函数= [file_ballback_handler]
)
return multi_route_chain
最后,它包含一个允许用户交互的main方法:
如果__name__ == "__main__":
#将您的API密钥放在这里或在您的环境中定义它
# os.environ["OPENAI_API_KEY"] = ''
prompt_info, destination_chains, default_chain = generate_destination_chains()
链= generate_router_chain(prompt_info, destination_chains, default_chain)
with open('conversation.log', 'w') as f:
while True:
question = prompt(
HTML("Type Your question ('q' to exit, 's' to save to html file): ")
)
if question == 'q':
break
如果['s', 'w']中的疑问:
file_ballback_handler.create_html()
continue
result = chain.run(question)
f.写(f”问:{问题}\ n \ n”)
f.write(f"A: {result}")
f.写(“\ n \ n ====================================================================== \ n \ n”)
print(result)
print()
Final Thoughts
LangChain允许创建真正复杂的llm交互流.
然而,设置工作流比想象的要复杂一些,因为 langchain.chains.router.MultiPromptChain 似乎不太合得来 langchain.chains.SimpleSequentialChain. 因此,我们需要创建一个自定义类来创建复杂的流.